Sobel Filters for Edge Detection
When it comes to edge detection, sobel filters are a powerful way to amplify the vertical and horizontal boundaries on an image.
Mathematically, sobel filters are represent as:
$$ sobel_x = \begin{bmatrix} -1 & 0 & 1\\ -2 & 0 & 2 \\ -1 & 0 & 1 \end{bmatrix} , sobel_y = \begin{bmatrix} -1 & -2 & -1\\ 0 & 0 & 0 \\ 1 & 2 & 1 \end{bmatrix} $$
In essence, these filters operate by taking a derivative of an image in both the $x$ and $y$ directions, thereby accentuating vertical and horizontal edges through changes in pixel intensity.
To demonstrate the impact of these filters, lets apply both $sobel_x$ and $sobel_y$ on an image of New York City.
import cv2
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
%config InlineBackend.figure_format = 'retina'
new_york = cv2.imread('new_york.jpg')
new_york = cv2.cvtColor(new_york, cv2.COLOR_BGR2RGB)
new_york_gray = cv2.cvtColor(new_york, cv2.COLOR_RGB2GRAY)
# Show images
fig, axs = plt.subplots(1, 2)
axs[0].imshow(new_york)
axs[0].axis('off')
axs[0].set_title('New York: Original')
axs[1].imshow(new_york_gray, cmap='gray')
axs[1].axis('off')
axs[1].set_title('New York: Gray Scale')
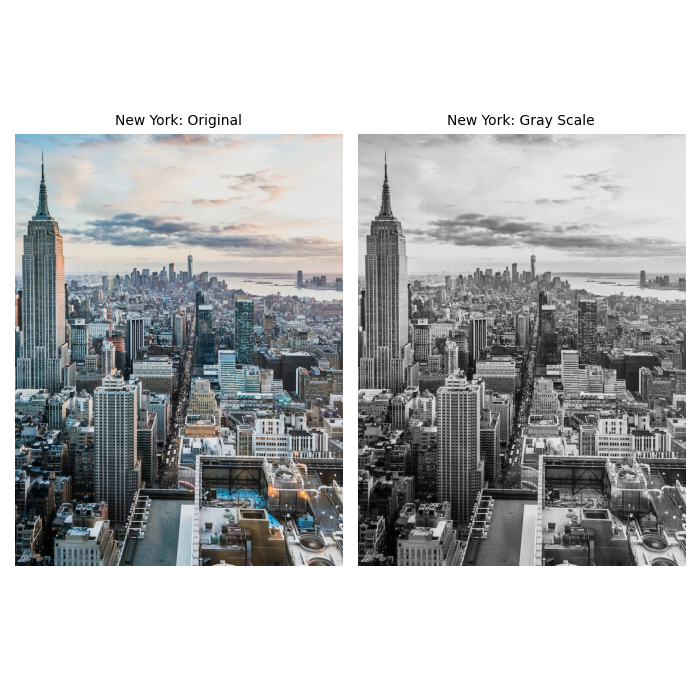
Applying Sobel Filters
OpenCV's filter2D() method allows us to apply defined filters to an image. Below, we define and apply both filters to the grayscale image
sobel_x = np.array([[ -1, 0, 1],
[ -2, 0, 2],
[ -1, 0, 1] ])
sobel_y = np.array([[ -1, -2, -1],
[ 0, 0, 0],
[ 1, 2, 1] ])
new_york_gray_x = cv2.filter2D(new_york_gray, -1, sobel_x)
new_york_gray_y = cv2.filter2D(new_york_gray, -1, sobel_y)
Visualize the outcome
fig, axs = plt.subplots(1, 2)
axs[0].imshow(new_york_gray_x, cmap='gray')
axs[0].axis('off')
axs[0].set_title('Sobel X: Vertical Edges')
axs[1].imshow(new_york_gray_y, cmap='gray')
axs[1].axis('off')
axs[1].set_title('Sobel Y: Horizontal Edges')
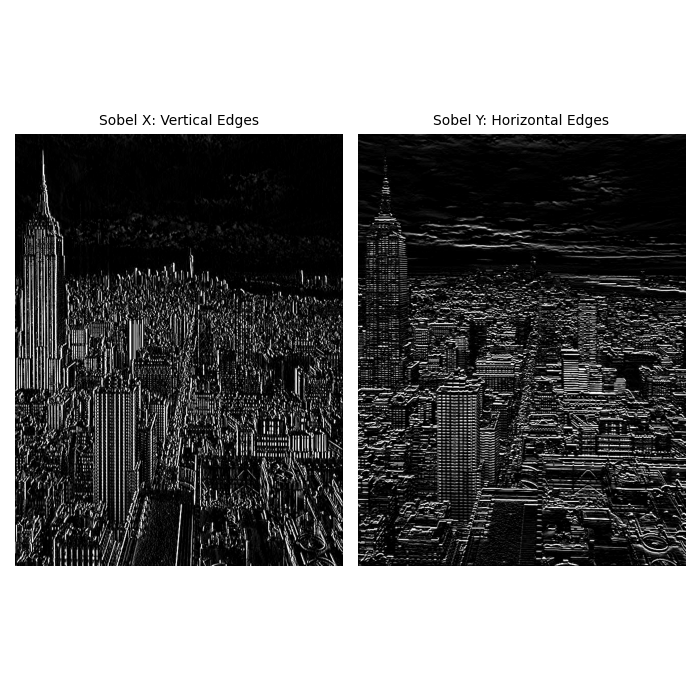
While on this example, we have applied the sobel filters independently and on the original image, in practice, it is often useful to apply a low-pass filter to reduce noise in an image before applying high-pass filters like the sobel filters in combination. This is part of what the Canny Edge detector does to find relevant edges on an image.