Adaptive Histogram Equalization
Histogram equalization uses all pixel values to improve the contrast of an image. While it can improve contrast through intensity distribution, it can sometimes fail, leading to overly bright images. This happens especially when the original image has a significant concentration of white and dark tonal intensities (or sharp contrast). To demonstrate this, let's implement histogram equalization on an image with a high-intensity difference.
import cv2
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
%config InlineBackend.figure_format = 'retina'
img = cv2.imread('eclipse.png')
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
# plot the image
plt.imshow(img)
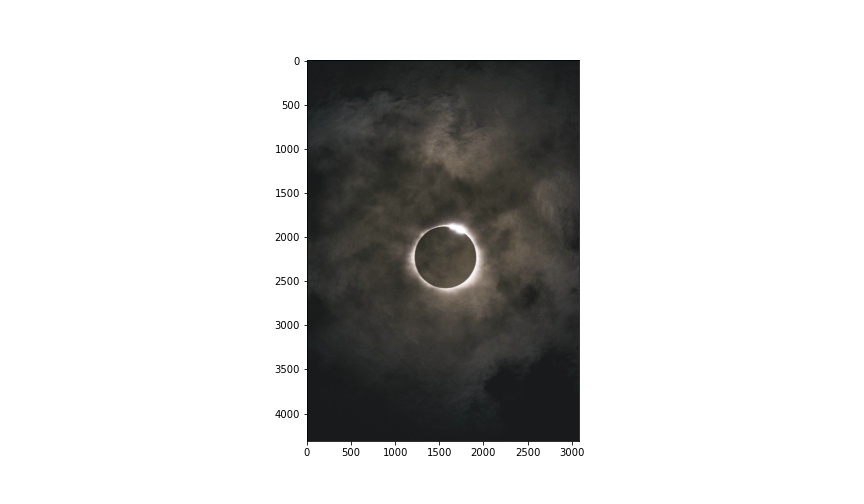
Implementing a histogram equalization on the image using the LAB color scheme.
def luminosity_equalization(input_img):
lab_img = cv2.cvtColor(input_img, cv2.COLOR_RGB2Lab)
l, a, b = cv2.split(lab_img)
eq_l = cv2.equalizeHist(l)
merged_img = cv2.merge([eq_l, a, b])
eq_img = cv2.cvtColor(merged_img, cv2.COLOR_LAB2RGB)
return eq_img
eq_img = luminosity_equalization(img)
fig = plt.figure(figsize=(14, 7))
fig.add_subplot(121)
plt.imshow(img)
plt.title('Original Image')
fig.add_subplot(122)
plt.imshow(eq_img)
plt.title('Histogram Equalized')
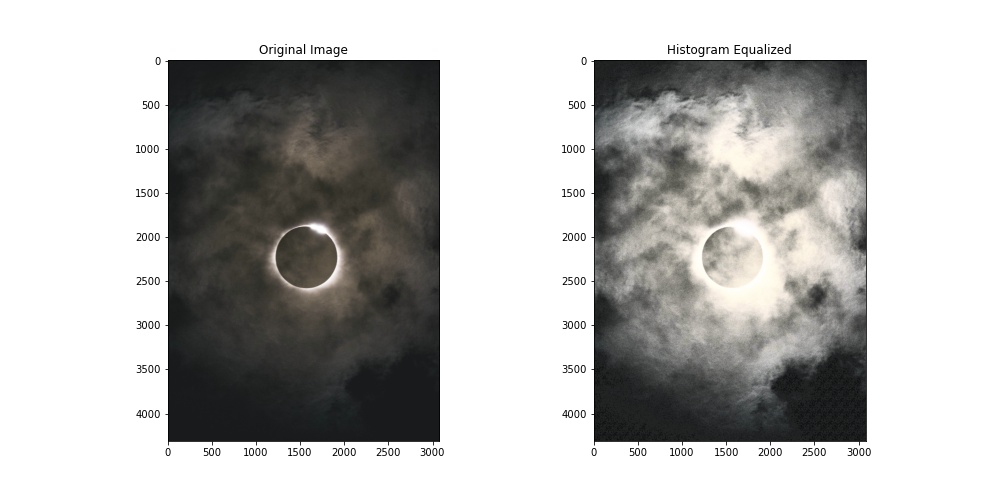
Notice that equalization has significantly brightened many parts of the image and that we have lost some contrast definition around the moon and the sum in comparison to the first image. A useful technique that improves contrast at a localized level is Adaptive Histogram Equalization.
CLAHE - Contrast Limiting Adaptive Histogram Equalization
Contrast Limit AHE is a variant of the AHE method that used clipping to preserve local contrast. Implementation-wise, the method uses the clipLimit to identify any pixels above that limit and distribute them equally to the rest of the block pixels before the equalization is performed. In other words, clipLimit is a threshold for contrast preservation of the local block size of the image.
OpenCV proves an easy way to implement CLAHE. To perform CLAHE operation, we call the createCLAHE method which takes on two arguments:
- 1. clipLimit: Threshold for local contrast preservation
- 2. tileGridSize: The size of the tile to compute histogram equalization.
CLAHE Implementation
def clahe_equalization(input_img, clip_limit):
# Convert image from BGR to LAB
lab_img = cv2.cvtColor(input_img, cv2.COLOR_RGB2Lab)
# Split image into L, A, B channels
l, a, b = cv2.split(lab_img)
# Create clahe
clahe = cv2.createCLAHE(clipLimit=clip_limit, tileGridSize=(8,8))
eq_l = clahe.apply(l)
# Equalized Image
eq_image = cv2.cvtColor(cv2.merge([eq_l, a, b]), cv2.COLOR_Lab2RGB)
return eq_image
clahe_image = clahe_equalization(sample_image, clip_limit=4.0)
Visualizing CLA Equalization
fig = plt.figure(figsize=(20, 7))
fig.add_subplot(131)
plt.imshow(img)
plt.title('Original Image')
fig.add_subplot(132)
plt.imshow(eq_img)
plt.title('Histogram Equalized')
fig.add_subplot(133)
plt.imshow(clahe_image)
plt.title('CLAHE Equalized')
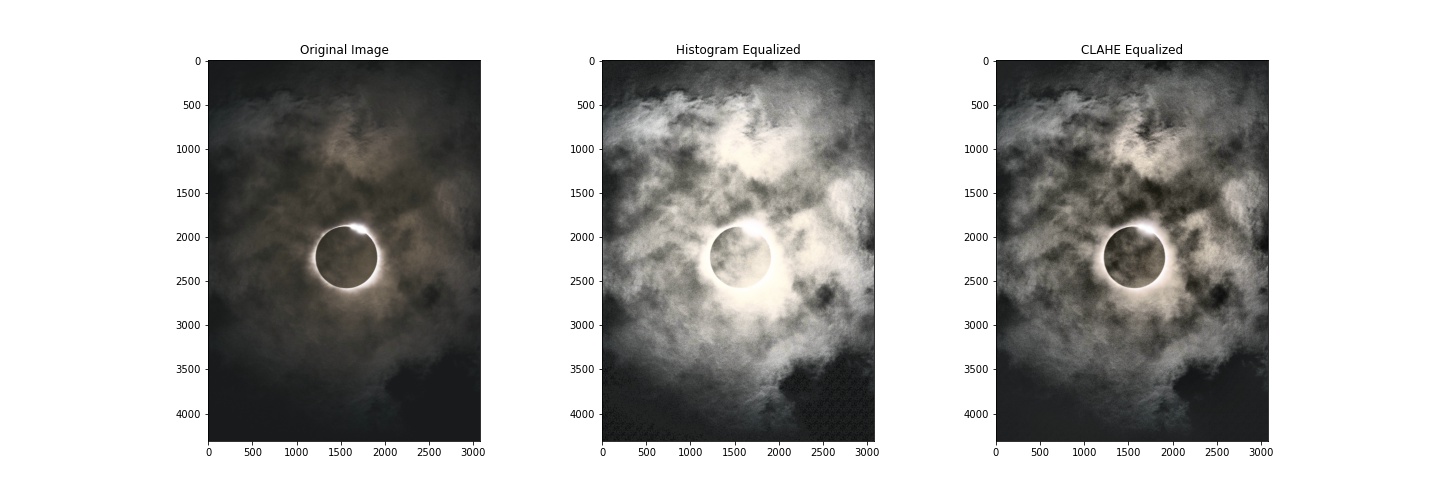