Haars Cascades Face Detection
Facial detection and recognition software has become a commonplace tool for security and access control. From unlocking mobile devices and accessing private spaces to self-checkouts at local stores, this technology is now a popular way to identify individuals and grant access to services.
One of the most widely-known algorithms for developing facial detection software is Haar Cascade. This machine learning-based approach involves a series of computations for different regions of an image to determine if the features in that area constitute a facial feature. These features could be anything from the eyes, smile, or even the entire face.
This note demonstrates Face Detection Implementation with Haars Cascade
Haar Cascades
Haar Cascade is a pre-trained algorithm used for object detection in images and videos, particularly for face detection. It is a machine learning-based approach that uses a set of Haar-like features to identify objects of interest in an image. These features are simple rectangular patterns that are used to describe the texture and contrast differences in an image
Haar Cascade has been widely used for face detection. It is fast and accurate, making it a popular choice for real-time applications. However, as we will see, it may perform poorly on complex features.
Sample Image for Detection
To set up this problem, I use an image with a few obvious faces and some not-so-obvious (i.e. occluded) faces to a computer to challenge the model.
import cv2
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
%config InlineBackend.figure_format = 'retina'
img = cv2.imread('kyle-smith-XMGaBN4fM58-unsplash.jpg')
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
img_gray = cv2.cvtColor(img, cv2.COLOR_RGB2GRAY)
# plot images side by side
fig = plt.figure(figsize=(25, 20))
fig.add_subplot(1, 2, 1)
plt.imshow(img)
plt.axis('off')
plt.title('Original Image')
fig.add_subplot(1, 2, 2)
plt.imshow(img_gray, cmap='gray')
plt.axis('off')
plt.title('Gray Scale Image')
plt.tight_layout()
Photo Credit: Photo by Kyle Smith on Unsplash
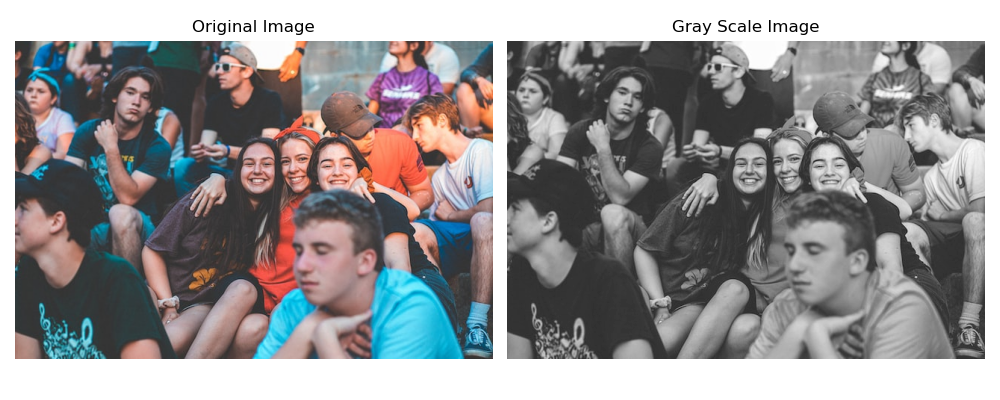
Implementing Haar Cascades Face Detection.
Haar Cascade models are located on the cv2.data.haarcascades path. For this note, I'll use the frontalface_default model to detect faces in the image. The implementation steps are straightforward:
- 1. Initialize the model with OpenCV.CascadeClassifier
- 2. Run detection by passing a grayscale image and parameters, $scale$ and $minNeigbor$
- 3.Visualizing Detected Faces using returned bounding boxes on the original image.
1. Initialize the model
To initialize the classifier, you can download any of the pre-trained models with the extension .xml. Alternatively, you can initialize them directly from opencv.
face_model = cv2.CascadeClassifier( f"{cv2.data.haarcascades}haarcascade_frontalface_default.xml" )
2. Running Detection on an Image
There are several methods that can be used for detection. In the example below, I use detectMultiScale, which takes in a gray_scale image, scale argument, and minNeigbor argument as parameters for detection.
detected_faces = face_model.detectMultiScale(img_gray, 1.2, 5)
detected_faces
array([[ 6, 51, 43, 43], [345, 139, 58, 58], [296, 141, 60, 60], [134, 50, 58, 58], [399, 141, 64, 64], [332, 235, 115, 115]], dtype=int32)
3. Visualizing Detected Faces
The detected_faces variable returns a list of coordinates for faces detected. We can then visualize the location of these faces by mapping the coordinates into the image as follows.
img_gray_copy = img_gray.copy()
for face in detected_faces:
x, y, h, w = face
cv2.rectangle(img_gray_copy, (x, y), (x+h, y+w), (255, 255, 255), 2)
cv2.rectangle(img, (x, y), (x+h, y+w), (255, 255, 255), 2)
fig = plt.figure(figsize=(25, 20))
fig.add_subplot(1, 2, 1)
plt.imshow(img)
plt.axis('off')
fig.add_subplot(1, 2, 2)
plt.imshow(img_gray_copy, cmap='gray')
plt.axis('off')
plt.tight_layout()
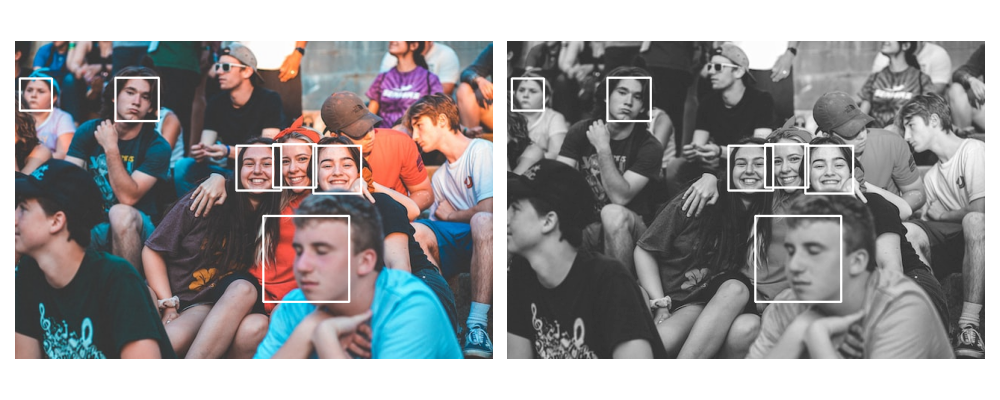
Out of the box, the model performs quite well for fully visible faces. However, half faces and faces that are occluded are not recognized. Other techniques can be used to further develop the model to detect mode faces.